Registering an ENS domain and setting up reverse lookup
Ethereum Name Service, or ENS for short, offers an Ethereum-based, decentralized alternative to domain management. In this short article we’ll discuss registering a domain and setting up reverse lookup for your newly registered domain.
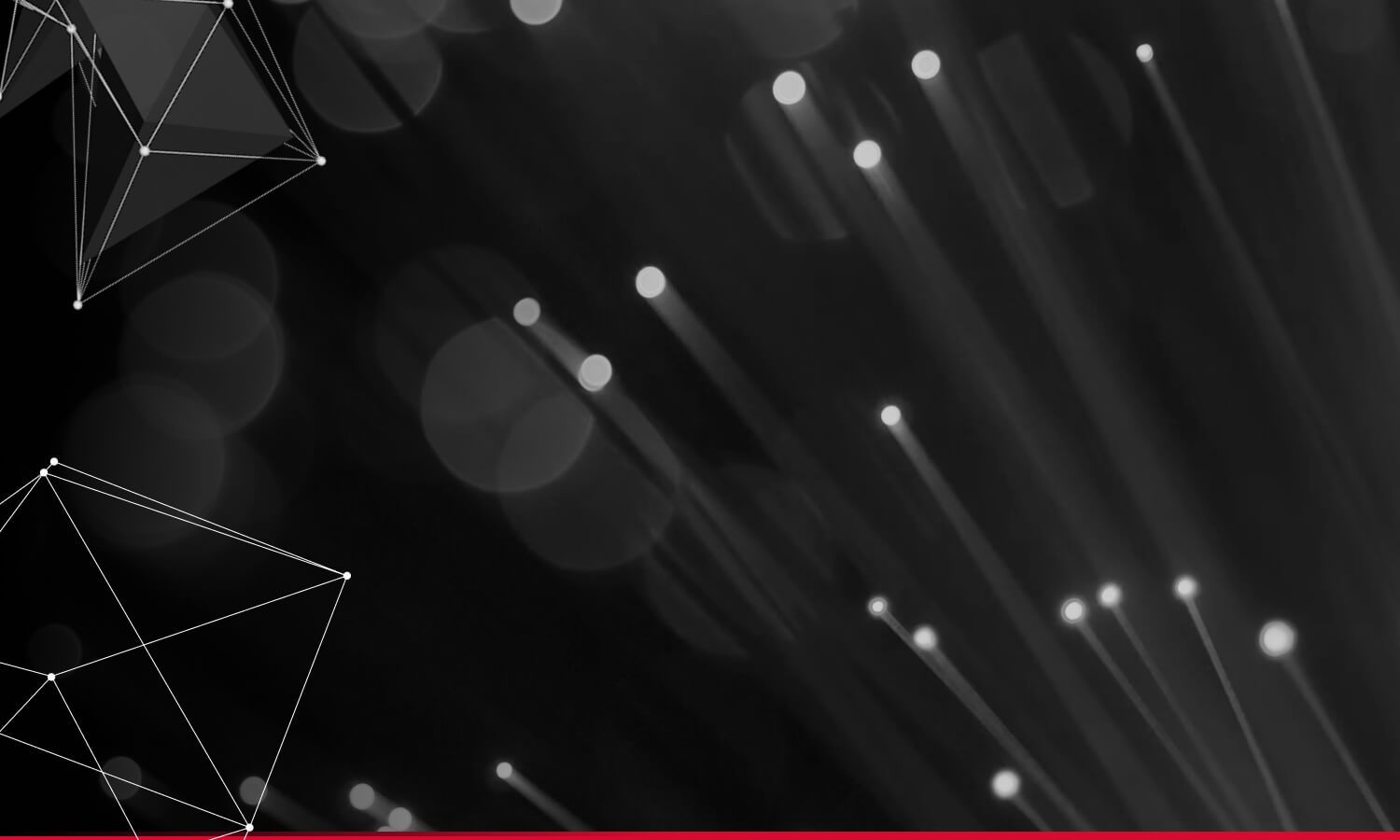
Ethereum Name Service, or ENS for short, offers an Ethereum-based, decentralized alternative to domain management. In this short article we’ll discuss registering a domain and setting up reverse lookup for your newly registered domain.
This solution allows you to set up a canonical name for your Ethereum address. Think of it like a user accessing your website through your IP address and you letting them know they can do it through “www.mycoolwebsite.biz”.
We’ll go through the whole process using the Ropsten test network, while describing some differences compared to Mainnet at the end.
The complete code is available over at GitHub. We’ll skip some boilerplate in the article.
The ENS registry on Ropsten is deployed at 0x112234455c3a32fd11230c42e7bccd4a84e02010
. Ropsten offers two TLDs: .eth
(standard auction-based registry) & .test
(domains for testing, with free registration where ownership expires after 4 weeks). We’ll focus on the .test
TLD in this article.
Let’s grab the registry address from the ENS contract and instantiate the registry contract:
const registrarAddress = await ens.methods.owner(namehash('test')).call()
// 0x21397c1A1F4aCD9132fE36Df011610564b87E24b
const registrar = new web3.eth.Contract(registrarAbi, registrarAddress);
I’ll then register a domain, let’s say nikola.test
:
await registrar.methods.register(web3.utils.sha3("nikola"), myAccount).send({from: 'myAccount'})
At this point you’ve acquired your domain. You can verify this by calling await ens.methods.owner(namehash(‘nikola.test')).call()
which will return your address.
Next, you’ll probably want to set up a resolver address for it.
Resolvers contain actual data for domains (like DNS records do). The default implementation contains various metadata and it can be extended further to contain additional records without breaking compatibility (again, just like DNS). For example, we’ve previously deployed a resolver extended with MX records for Melon Mail. You don’t need to do this to set up reverse lookup but your domain doesn’t do much without it.
Setting up a resolver is as simple as calling:
await ens.methods.setResolver(namehash(‘nikola.test’), resolverAddress).send({from: myAccount})
Again easily verifiable with:
await ens.methods.resolver(namehash('nikola.test')).call()
We’ll focus on setting up reverse lookup for our domain. Reverse lookup simply means getting an ENS domain from an Ethereum address. This is done using a special TLD addr.reverse.
You might think this is as simple as a reverse mapping on the contract, but keep in mind you can have two or more domains pointing to the same address, so a 1-1 mapping isn’t possible.
Instead, a special addr.reverse
TLD is created. The actual reverse lookup process is the same as the traditional lookup process but for the domain yourAccount.addr.reverse
(yourAccount without the 0x
prefix).
You’ll need to use the reverseResolver contract for this, which is actually the owner of the addr.reverse
TLD.
const reverseRegistarAddr = await ens.methods.owner(namehash('addr.reverse')).call()
// 0x67d5418a000534a8F1f5FF4229cC2f439e63BBe2
const reverseRegistrar = new web3.eth.Contract(reverseRegistrarAbi, reverseRegistarAddr)
The reverse registrar acts as a proxy here, using its protected resolver to manage *.addr.reverse
domains, but allowing you to manage the one with your address. We’ll call setName
on the reverse registrar which will set the resolver for yourAccount.addr.reverse
to the reverse registrar’s protected resolver and set it to point to our nikola.test
domain.
await reverseRegistrar.methods.setName('nikola.test').send({ from: yourAccount })
We can go through the reverse lookup process to verify this:
await ens.methods.resolver(namehash('11b6c106d52f0bb8adb75a577076d33f33fa9c40.addr.reverse')).call()
// 0x53350F4089B10E516c164497f395Dbbbc8675e20 - defaultResolver from reverseResolver
await publicResolver2.methods.name(namehash(yourAccount.substr(2) + '.addr.reverse')).call()
// nikola.test
You are now (hopefully, if you’ve made it this far) a proud owner of a .test
ENS domain that can be looked up from your Ethereum address. Congratulations and thanks for bearing with me!
The process on Mainnet is actually pretty similar. The biggest difference is in acquiring an .eth domain, which is auction based and a special topic on its own. Also, managing the domain should be much easier with sites like MyEtherWallet providing an interface to the contracts.
Anyway,
Cool, right? 😎